Improving User Interface Responsiveness in iOS Apps
In iOS development, a responsive user interface (UI) isn’t merely a nicety—it’s essential to user retention, app performance, and an overall polished user experience. Responsiveness refers to the app’s ability to react swiftly to user input, provide feedback in real time, and maintain fluidity even under heavy loads. With iOS app users expecting snappy interactions, unresponsiveness can quickly lead to frustration and app abandonment. Here, we’ll explore proven strategies to boost UI responsiveness in iOS apps, focusing on performance optimizations, efficient thread management, and best practices for UI updates.
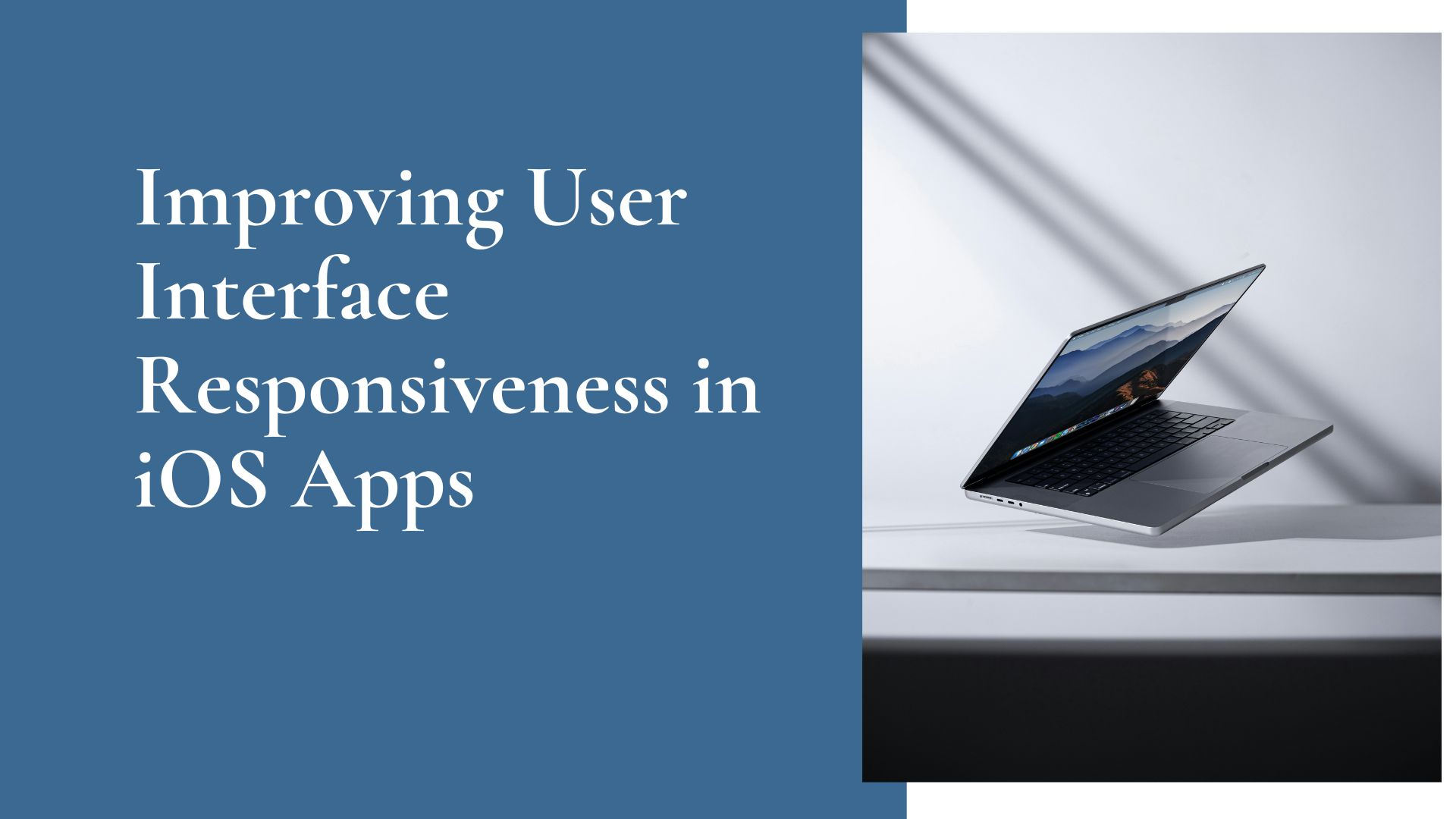
1. Optimize Main Thread Usage
The main thread is where all UI updates occur in iOS. Overloading it with non-UI tasks is one of the most common causes of sluggishness. Ensuring that resource-intensive processes are offloaded from the main thread can make a substantial difference.
Use Grand Central Dispatch (GCD): GCD makes it straightforward to send tasks to background threads. For example, using
DispatchQueue.global()
to perform network or heavy data operations prevents the main thread from stalling. Always bring UI updates back to the main queue withDispatchQueue.main.async
to keep UI-related tasks and view updates synchronized.Leverage NSOperationQueue: For more complex thread handling or dependencies, NSOperationQueue provides more control, such as prioritizing tasks and setting dependencies, which can be invaluable for managing complex background work.
Adopt Asynchronous Patterns: Use async/await with Swift concurrency to handle asynchronous code more readably and efficiently, especially for iOS 15 and later. Structured concurrency minimizes the risk of creating “callback hell” and simplifies background processing by allowing the system to manage thread lifecycles intelligently.
2. Use Lazy Loading and View Recycling
Reducing the initial load time for complex views can significantly enhance perceived responsiveness.
Lazy Loading: Avoid loading all data and views at once. Load only the views visible on the screen and gradually populate others as the user scrolls. This approach is crucial for large datasets or images, as loading too much data upfront can slow down UI performance.
Reuse Cells in Lists: When working with
UITableView
orUICollectionView
, make sure cells are efficiently dequeued and reused. Swift’s Diffable Data Source, introduced in iOS 13, makes managing these cells easier and reduces the likelihood of duplicate views, resulting in faster loading times for dynamic lists.On-Demand Image Loading: Download and render images asynchronously, especially in lists. Libraries like SDWebImage can help by caching and asynchronously loading images to ensure they don’t block the main thread.
3. Efficiently Handle Large Data Sets
Handling large amounts of data on the main thread can result in noticeable UI delays, so it’s crucial to use efficient methods.
Use Core Data with Background Contexts: If your app depends on a Core Data stack, work with background contexts to fetch and save data without interrupting UI interactions. Since iOS 10, Core Data has supported background contexts natively, so perform heavy data operations outside the main context and merge them into the main context when necessary.
Implement Pagination or Infinite Scrolling: Rather than loading all data at once, use pagination techniques to load data incrementally. This approach reduces the amount of memory and CPU power needed at any given time, making the app feel smoother.
Cache Strategically: Use caching mechanisms to store frequently accessed data, reducing load times for views that need data frequently. NSCache or even lightweight databases like SQLite for caching can help retain data without reloading, especially in resource-intensive or data-heavy applications.
4. Optimize Animations for Smoothness
Animations are a powerful UI tool, but poorly implemented animations can degrade responsiveness, creating a choppy or unresponsive feel.
Use Core Animation: Core Animation is optimized for the iOS GPU, making it ideal for smooth animations. Aim to move most of the animation work to Core Animation layers (
CALayer
) rather than relying on custom draw calls or view hierarchy changes that may overuse the CPU.Minimize Layout Passes During Animations: Heavy layout recalculations during animations are a common performance pitfall. Leverage Auto Layout constraints efficiently, and avoid unnecessary layout changes to keep animations fluid.
Use UIKit Dynamics and Motion Effects Sparingly: While UIKit dynamics and motion effects add visual appeal, they can drain resources if overused. Optimize these effects to avoid impacting the smoothness of primary interactions.
5. Leverage Instrumentation for Performance Monitoring
Performance analysis tools allow you to pinpoint bottlenecks effectively.
Instruments App: Use Instruments (part of Xcode) to profile CPU usage, memory usage, and thread usage. The “Time Profiler” instrument is particularly useful for identifying which parts of your code are consuming the most resources. The “Main Thread Checker” tool is invaluable for catching off-main-thread UI updates, which can cause delays and crashes.
View Debugging: The View Debugging tool in Xcode helps visualize your view hierarchy, making it easier to spot redundant views or large view hierarchies that can slow down responsiveness. A streamlined hierarchy minimizes layout recalculations and improves response times.
Memory Management with Leaks Instrument: Efficient memory management is essential for responsiveness. Use the Leaks instrument to check for memory leaks that could lead to app slowdowns or crashes. Eliminating memory leaks, especially in views that are frequently updated, is critical for smooth performance.
6. Adopt the Latest iOS Frameworks
Apple frequently introduces frameworks and updates designed to optimize app performance.
SwiftUI: SwiftUI simplifies UI updates, minimizes the view hierarchy, and offers smooth declarative syntax for animations. While it’s still evolving, SwiftUI can improve responsiveness, especially for dynamic interfaces with frequent updates. Its automatic view invalidation mechanism reduces the chance of unnecessary UI updates.
Combine Framework: Combine allows you to manage data flow and handle asynchronous events effectively. Using Combine, you can consolidate various sources of UI updates (like network responses or database changes) into a single, efficient flow, minimizing potential UI bottlenecks.
AsyncImage in SwiftUI: For iOS 15 and later,
AsyncImage
is an efficient way to load images asynchronously, reducing the main thread load, and contributing to faster image loading within UI.
7. Focus on Feedback and Perceived Performance
Improving UI responsiveness isn’t just about the actual performance but also how responsive the app feels.
Use Activity Indicators and Loading Placeholders: Implement activity indicators or skeleton views to indicate loading times. These feedback mechanisms let users know the app is processing, which reduces the perception of delay. For example, using a subtle loading spinner while data loads or displaying a skeleton screen until actual content appears can keep users engaged.
Defer Non-Critical Operations: Break down and defer non-essential tasks, so that users experience the primary UI functions without delay. For instance, delay analytics updates or data syncing until after the user interaction has completed to keep the UI feeling snappy.
Implement Haptic Feedback: Haptic feedback gives users immediate, tactile responses, which can make actions feel more responsive, especially on devices with haptic engines. While it doesn’t technically enhance performance, it improves the overall user experience.
Conclusion: Building for Smooth, Responsive Interactions
Creating a highly responsive UI in iOS apps requires a multifaceted approach, involving careful use of threads, efficient data handling, streamlined animations, and ongoing performance monitoring. By following these practices, developers can significantly improve responsiveness, delivering a seamless user experience. As iOS and Apple’s frameworks continue to evolve, adopting new technologies and refining optimization techniques is essential to building apps that not only perform well today but are also prepared for future advancements.
With a responsive UI, users will not only engage more with your app but also trust it to be an efficient, enjoyable part of their mobile experience.